Deep neural network on MNIST using Keras#
The first layer in this case is not actually a layer of neurons but a Flatten function to flatten the 28 x 28 image into a single array of 784, which is fed to the first hidden layer of 50 neurons. The last layer has 10 neurons corresponding to the 10 classes with a softmax activation function.
1raise SystemExit("Stop right there!");
An exception has occurred, use %tb to see the full traceback.
SystemExit: Stop right there!
Importing libraries and packages#
1# System
2import os
3
4# Modelling
5import tensorflow as tf
6from tensorflow.keras.models import Sequential
7from tensorflow.keras.layers import Dense
8from tensorflow.keras.layers import Flatten
9
10# Plotting
11import matplotlib.pyplot as plt
12
13%matplotlib inline
1os.environ["TF_CPP_MIN_LOG_LEVEL"] = "2"
Loading dataset#
The MNIST dataset is integrated into the TensorFlow library. It consists of 70,000 handwritten images of the digits 0 to 9.
1# Creating an instance of the MNIST dataset
2dataset = tf.keras.datasets.mnist
3# Loading the MNIST dataset's train and test data
4(train_features, train_labels), (
5 test_features,
6 test_labels,
7) = dataset.load_data()
Training of the network#
1# Normalizing the data
2train_features, test_features = train_features / 255.0, test_features / 255.0
1# Build the sequential model
2model = Sequential()
3# The first layer in this case is not actually a layer of
4# neurons but a Flatten function
5model.add(Flatten(input_shape=(28, 28)))
6model.add(Dense(units=50, activation="relu"))
7model.add(Dense(units=20, activation="relu"))
8model.add(Dense(units=10, activation="softmax"))
For a multiclass classifier, the following loss functions are used: sparse_categorical_crossentropy, which is used when the labels are not one-hot encoded, as in this case; and, categorical_crossentropy, which is used when the labels are one-hot encoded.
1# Providing training parameters using the compile method
2model.compile(
3 optimizer="adam",
4 loss="sparse_categorical_crossentropy",
5 metrics=["accuracy"],
6)
1# Inspecting the model configuration using the summary function
2model.summary()
Model: "sequential"
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
flatten (Flatten) (None, 784) 0
_________________________________________________________________
dense (Dense) (None, 50) 39250
_________________________________________________________________
dense_1 (Dense) (None, 20) 1020
_________________________________________________________________
dense_2 (Dense) (None, 10) 210
=================================================================
Total params: 40,480
Trainable params: 40,480
Non-trainable params: 0
_________________________________________________________________
1# Train the model by calling the fit method
2model.fit(train_features, train_labels, epochs=50)
Epoch 1/50
1875/1875 [==============================] - 8s 4ms/step - loss: 0.6027 - accuracy: 0.8255
Epoch 2/50
1875/1875 [==============================] - 12s 6ms/step - loss: 0.1733 - accuracy: 0.9509
Epoch 3/50
1875/1875 [==============================] - 17s 9ms/step - loss: 0.1216 - accuracy: 0.9642
Epoch 4/50
1875/1875 [==============================] - 13s 7ms/step - loss: 0.0962 - accuracy: 0.9710
Epoch 5/50
1875/1875 [==============================] - 12s 6ms/step - loss: 0.0778 - accuracy: 0.9769
Epoch 6/50
1875/1875 [==============================] - 15s 8ms/step - loss: 0.0632 - accuracy: 0.9800
Epoch 7/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0578 - accuracy: 0.9831
Epoch 8/50
1875/1875 [==============================] - 13s 7ms/step - loss: 0.0498 - accuracy: 0.9844
Epoch 9/50
1875/1875 [==============================] - 12s 6ms/step - loss: 0.0463 - accuracy: 0.9853
Epoch 10/50
1875/1875 [==============================] - 8s 4ms/step - loss: 0.0422 - accuracy: 0.9866
Epoch 11/50
1875/1875 [==============================] - 12s 7ms/step - loss: 0.0358 - accuracy: 0.9892
Epoch 12/50
1875/1875 [==============================] - 16s 8ms/step - loss: 0.0332 - accuracy: 0.9899
Epoch 13/50
1875/1875 [==============================] - 13s 7ms/step - loss: 0.0291 - accuracy: 0.9908
Epoch 14/50
1875/1875 [==============================] - 15s 8ms/step - loss: 0.0281 - accuracy: 0.9906
Epoch 15/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0250 - accuracy: 0.9919
Epoch 16/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0242 - accuracy: 0.9924
Epoch 17/50
1875/1875 [==============================] - 8s 4ms/step - loss: 0.0217 - accuracy: 0.9929
Epoch 18/50
1875/1875 [==============================] - 13s 7ms/step - loss: 0.0205 - accuracy: 0.9935
Epoch 19/50
1875/1875 [==============================] - 7s 4ms/step - loss: 0.0168 - accuracy: 0.9945
Epoch 20/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0161 - accuracy: 0.9945
Epoch 21/50
1875/1875 [==============================] - 6s 3ms/step - loss: 0.0160 - accuracy: 0.9944
Epoch 22/50
1875/1875 [==============================] - 6s 3ms/step - loss: 0.0152 - accuracy: 0.9951
Epoch 23/50
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0142 - accuracy: 0.9952
Epoch 24/50
1875/1875 [==============================] - 8s 4ms/step - loss: 0.0137 - accuracy: 0.9952
Epoch 25/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0153 - accuracy: 0.9948
Epoch 26/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0124 - accuracy: 0.9958
Epoch 27/50
1875/1875 [==============================] - 15s 8ms/step - loss: 0.0113 - accuracy: 0.9962
Epoch 28/50
1875/1875 [==============================] - 20s 11ms/step - loss: 0.0103 - accuracy: 0.9966
Epoch 29/50
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0114 - accuracy: 0.9962
Epoch 30/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0101 - accuracy: 0.9969
Epoch 31/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0099 - accuracy: 0.9964
Epoch 32/50
1875/1875 [==============================] - 13s 7ms/step - loss: 0.0086 - accuracy: 0.9969
Epoch 33/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0085 - accuracy: 0.9971
Epoch 34/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0095 - accuracy: 0.9968
Epoch 35/50
1875/1875 [==============================] - 12s 6ms/step - loss: 0.0067 - accuracy: 0.9981
Epoch 36/50
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0096 - accuracy: 0.9966
Epoch 37/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0090 - accuracy: 0.9972
Epoch 38/50
1875/1875 [==============================] - 14s 7ms/step - loss: 0.0065 - accuracy: 0.9974
Epoch 39/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0074 - accuracy: 0.9975
Epoch 40/50
1875/1875 [==============================] - 18s 10ms/step - loss: 0.0075 - accuracy: 0.9974
Epoch 41/50
1875/1875 [==============================] - 12s 6ms/step - loss: 0.0090 - accuracy: 0.9968
Epoch 42/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0069 - accuracy: 0.9975
Epoch 43/50
1875/1875 [==============================] - 12s 6ms/step - loss: 0.0068 - accuracy: 0.9976
Epoch 44/50
1875/1875 [==============================] - 10s 5ms/step - loss: 0.0063 - accuracy: 0.9979
Epoch 45/50
1875/1875 [==============================] - 9s 5ms/step - loss: 0.0083 - accuracy: 0.9974
Epoch 46/50
1875/1875 [==============================] - 12s 7ms/step - loss: 0.0060 - accuracy: 0.9980
Epoch 47/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0071 - accuracy: 0.9977
Epoch 48/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0053 - accuracy: 0.9984
Epoch 49/50
1875/1875 [==============================] - 11s 6ms/step - loss: 0.0040 - accuracy: 0.9986
Epoch 50/50
1875/1875 [==============================] - 12s 6ms/step - loss: 0.0070 - accuracy: 0.9974
<tensorflow.python.keras.callbacks.History at 0x7f72449382b0>
Statistics#
1# Test the model by calling the evaluate() function
2model.evaluate(test_features, test_labels)
313/313 [==============================] - 1s 3ms/step - loss: 0.2188 - accuracy: 0.9728
[0.21879249811172485, 0.9728000164031982]
Predicting#
1loc = 100
2test_image = test_features[loc]
1test_image.shape
(28, 28)
1# The model expects 3-dimensional input
2test_image = test_image.reshape(1, 28, 28)
1result = model.predict(test_image)
2print(result)
[[4.7161498e-12 3.0817436e-16 5.4286241e-13 5.7867281e-20 3.8419296e-21
8.6845771e-15 1.0000000e+00 3.1443599e-16 2.3115226e-14 9.3220094e-26]]
1result.argmax()
6
1test_labels[loc]
6
1plt.imshow(test_features[loc])
<matplotlib.image.AxesImage at 0x7f722454e550>
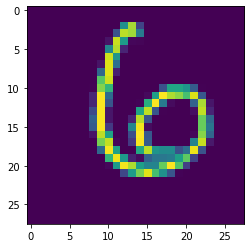